The
CODE
command compiles the Sprites and creates a set of assembly 65c816
Source Files
(Merlin 16+ compatible), one per Sprite :
Spr_010
CLC
; 36x62, 1483 bytes, 2570 cycles
SEI
;
Disable Interrupts
PHD
;
Backup Direct Page
TSC
; Backup Stack
STA
StackAddress
LDAL
$E1C068 ; Direct Page and Stack
in Bank 01/
ORA
#$0030
STAL
$E1C068
TYA
; Y =
Sprite Target Screen Address (upper left corner)
TCS
; New
Stack address
LDX
#$2222 ; Pattern #1 : 78
LDY
#$0000 ; Pattern #2 : 78
LDA
#$2200 ; Pattern #3 : 19
TCD
*--
TYA
; Line 0
STA
$A8,S
SEP
#$20
LDA
$04,S
AND
#$0F
ORA
#$10
STA
$04,S
LDA
$A4,S
AND
#$0F
...
*--
LDAL $E1C068 ;
Direct Page and Stack in Bank 00/
AND #$FFCF
STAL $E1C068
LDA
StackAddress ; Restore Stack
TCS
PLD
; Restore Direct Page
CLI
;
Enable Interrupts
RTL
|
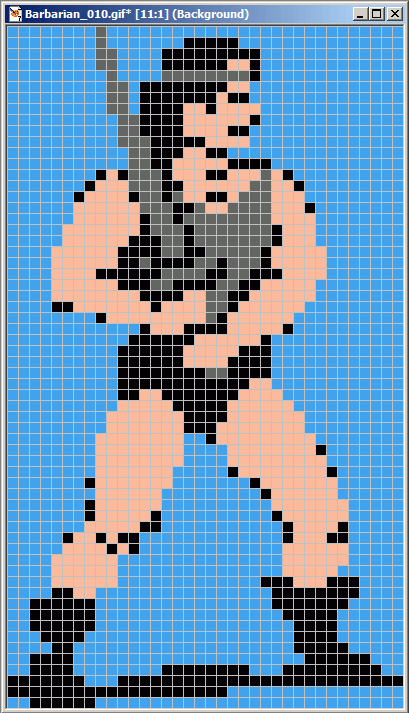 |
The source code file can be put in a Merlin 16+ project and be called
by a JSL.
The comments contain information such as Sprite Width / Height, Object
code Size, Number of Cycles used to display it...
Most
of the time, you don't care about manipulating ONE sprite, what you
want is to call a unique function dealing with all the sprites you have
in your game. To create this single entry point, use the command BANK
to generate both object code files (*.bin) and the single entry point
code (.txt) :
The
object code files are 64 KB long (fit in one memory bank).
The
latest one can be smaller than 64 KB. Such object files contain the
assembled version of the Source code provided by the CODE command. A
short code is provided as a Merlin 16+ compatible source file :
DrawBarb
ASL
;
A=Sprite Number ($0000-$0041)
TAX
; Y=Target
Screen Address
($2000-$9D00)
LDA
BarbNum,X ; Relative Sprite
Number Table
JMP (BarbBank,X) ;
Bank Number Table
BarbNum
HEX
1800,0500,2000,1C00,0000,2C00,1300,1400
HEX
0B00,0700,0900,0A00,1900,1A00,0900,0A00
HEX
1B00,1D00,2800,2A00,2600,2B00,0C00,0E00
HEX
2100,2200,1F00,1E00,1100,1400,2900,2400
HEX
0600,0200,0E00,0F00,1500,1000,1200,1100
HEX
0400,0500,0300,0200,0400,2300,1000,1300
HEX
0700,0800,2700,2500,0D00,0C00,0800,0600
HEX
0D00,0B00,0300,0100,0100,0000,1600,1700
HEX 0F00,1200
BarbBank
DA
BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank01,BarbBank01
DA
BarbBank01,BarbBank01,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank01,BarbBank01
DA
BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00
DA
BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00
DA
BarbBank00,BarbBank01,BarbBank01,BarbBank01,BarbBank00,BarbBank01,BarbBank01,BarbBank01
DA
BarbBank01,BarbBank01,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank00
DA
BarbBank00,BarbBank00,BarbBank00,BarbBank00,BarbBank01,BarbBank01,BarbBank01,BarbBank01
DA
BarbBank00,BarbBank00,BarbBank01,BarbBank00,BarbBank01,BarbBank01,BarbBank00,BarbBank00
DA BarbBank00,BarbBank00
BarbBank00 JSL
$AA0000
PHK
PLB
RTS
BarbBank01 JSL
$AA0000
PHK
PLB
RTS
With
a single entry point (DrawBarb),
the source code has to be included in your project. The DrawBarb function is
called with only two parameters : A
= Sprite identification number (see WallPaper for sprite
identification) and Y
= Target Screen address). It will find the right Bank and will
display the Sprite in the $01/2000 Graphic Page (shadowing On). As a
programmer, the only thing to do is to allocate the number of required
memory banks (= number of object code files), load the object files in
the memory banks and populate the BarBankXX JSL $AA0000
address with right ones.