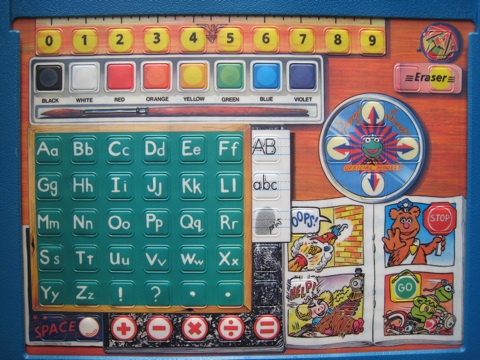
I believe it would be interesting to understand the inners of the keyboard. You just have to plug it into the joystick port and voilĂ ! But that has not been so easy as I did not have any software to communicate with the keyboard...
The learning keyboard is divided into 9 rows and 12 columns. Each key is dedicated to a specific "real" key: letters (both uppercase and lowercase), numbers, colors, computations (plus qnd minus signs, multiply, divide, equal sign) and function keys (erase, cancel, validate, stop, help, etc.)
Row 1: 0 1 2 3 4 5 6 7 8 9 # Zap Row 2: Black White Red Orange Yellow Green Blue Violet # # Eraser Eraser Row 3: # # # # # # # # # Up # # Row 4: A B C D E F Uppercase # Left Kermit Right # Row 5: G H I J K L Lowercase # # Down # # Row 6: M N O P Q R Print Oops # # # Stop Row 7: S T U V W X Unknown # # # # # Row 8: Y Z ! ? , . Unknown Help # # Go # Row 9: Space Space + - * / = # # # # #
The fastest way to understand the way it works is to create a simple BASIC program and press the different keys of the keyboard.
10 PRINT PDL(0), PDL(1) 20 REM 30 GOTO 10
The X and Y coordinates change based on the key
pressed. When a key is pressed, the X/Y address is set to
the joystick softswitches $C064 (X axis) $C065 (Y axis)
which can then be read by a program.
- Keys on the X-axis are every ~14 pixels
- Keys on the Y-axis are every ~18 pixels
- The uppper-left key (0) is at X=6, Y=8
- The possible X-values are between 6 and 160
- The possible Y-values are between 8 and 138
Now, let's write some code to communicate with the keyboard and collect the X/Y coordinates of the key pressed:
* * Jim Henson's Muppet Learning Keys * * (c) 1984, Henson Associates Inc. * (s) 2007, Antoine Vignau * mx %11 org $2000 lst off *-------------------------------------- joyBTN = $fd joyX = $fe joyY = $ff *-------------------------------------- jsr $fc58 ]lp jsr readJOY lda joyX jsr getXaxis jsr $fdda lda #" " jsr $fded lda joyY jsr getYaxis jsr $fdda lda #$8d jsr $fded lda $c000 bpl ]lp bit $c010 rts *-------------------------------------- Key to Axis * * The following routine returns * - for the X axis: 0 to B * - for the Y axis: 0 to 8 getXaxis cmp #$48 bcc getYaxis clc adc #$0a getYaxis and #$f0 lsr lsr lsr lsr rts *-------------------------------------- Lecture joystick readJOY php sei lda $c061 and #$80 sta joyBTN readJOY1 lda $c036 pha and #$7f sta $c036 ldx #128 ldy #0 lda $c070 ]lp lda $c064 bpl readJOY2 xba xba iny iny readJOY2 dex bne ]lp sty joyX ldx #128 ldy #0 lda $c070 ]lp lda $c065 bpl readJOY3 xba xba iny iny readJOY3 dex bne ]lp sty joyY pla sta $c036 plp rts
The code above is IIgs compliant but must be rewritten for other 8-bit Apple IIs.
Antoine, June 2008